Valid inequalities
Valid inequalities are additional constraints that can be added to a correct integer program that potentially strengthen the formulation, i.e. reduce the size of the feasible region for the linear programming relaxation.
For example, in the 0-1 knapsack problem,
for any set such that
\[ \sum_{i \in C} w_i > b \]we can add the cover inequalities
\[ \sum_{i \in C} x_i \leq |C|-1 \]To be completely concrete, suppose that , and our instance was
\[ \begin{aligned} &\max& x_1 + 2x_2\\ &\text{subject to}& 3x_1 + 4 x_2 &\leq 5\\ &&x_1,\, x_2 & \in \{0,1\}. \end{aligned} \]The feasible region of this LP and the feasible region of the integer hull are shown below.
Observing that , we can apply a cover inequality with the set to obtain the inequality
\[ x_1 + x_2 \leq 1. \]Observe that adding this inequality to the knapsack formulation makes the LP relaxation integral, thus it is a stronger formulation.
User Cuts in CPLEX
User cuts are, at a high level, valid inequalities for an integer program CPLEX will optionally add while searching for an integer solution. Like lazy cuts, user cuts can be added either before the optimization begins (with addUserCut()
from IloCplex
) or by using a UserCutCallback
(documentation here) to detect violated constraints on the fly and add them to the formulation. CPLEX checks for violated user cuts at the highlighted stage in the diagram below.
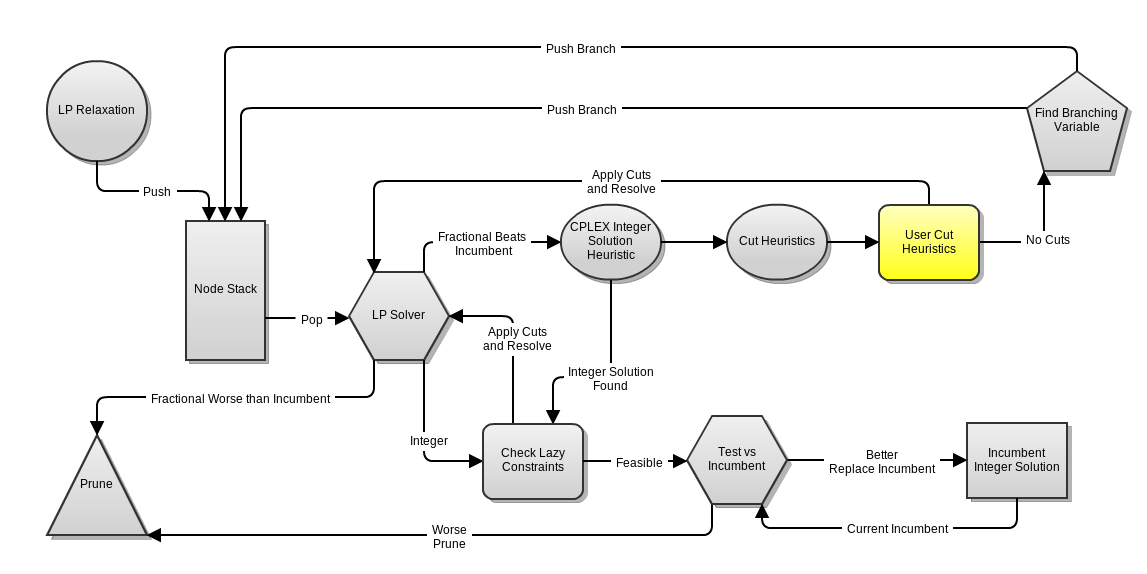
As you can see, there is no guarantee that a feasible solution will satisfy all of the user cuts, as LP solutions which are integral are sent through the lazy constraints to the incumbent. Thus if a constraint is necessary for the formulation to be correct, but you want to check for it as a user cut, you must also check for it as a lazy cut. Additionally, we have the benefit that our method for identifying user cuts doesn't need to work 100% of the time, as if it fails we always have the lazy cuts for insurance. This is how we will be using user cuts for our TSP solver.
Using UserCutCallback
UserCutCallback
works very similarly to LazyConstraintCallback
. Mathematically, you are passing CPLEX the following function:
- Input: A fractional solution to the LP relaxation of your problem, potentially after some lazy constraints, CPLEX generated cuts, or user cuts have been added
- Output: A list (potentially empty) of violated valid inequalities.
Note that there is no assumption that the list is is nonempty, and that there is no guarantee that the UserCutCallback
will ever be checked. The Javadoc for UserCutCallback
can be found here, but we summarize the important methods in the table below (the interface is very similar to that of LazyConstraintCallback
):
Method Name |
Return Type |
Arguments |
Description |
---|---|---|---|
getValue |
double |
IloNumVar var |
Returns the solution value of var at the current node. |
getValues |
double[] |
IloNumVar[] vars |
Returns the solution values for vars at the current node. |
add |
IloRange |
IloRange cut |
Adds the constraint cut to the model. Assumes cut is linear. |
getNnodes64 |
long |
|
Returns the total number of branch and bound nodes created thus far in the optimization. E.g. returns 0 at the root node. |
isAfterCutLoop |
boolean |
|
This method indicates whether or not the callback was invoked after CPLEX stopped generating cuts and called the callback a final time. |
The final method requires some explanation. The "cut loop" refers to the loop in the above diagram where CPLEX looks for cuts, adds cuts, and resolves the LP relaxation. While CPLEX could do this indefinitely until an optimal integer solution was found (which would solve the whole IP, see cutting plane method and chapter 9 of Optimization over the Integers), this would generally take a long time. Instead, CPLEX uses some internal Heuristics (which you can toggle, although I don't recommend it) to control how many cuts to add, then it "exits the cut loop" and will not generate more cuts. By calling isAfterCutLoop
, you can tell if CPLEX is done adding cuts before you start adding your own. It might be a good idea to wait until CPLEX is done, as the cuts it adds may change the LP so much that the cut you were about to add becomes irrelevant. Alternatively, the cut you add may cause CPLEX to add smarter cuts. Whether or not waiting until the cut loop is over to add cuts is something that you will need to determine for your own problem.
Otherwise, the same warnings as before about using cplex.ge()
instead of cplex.addGe()
and using getValues()
instead of getValue()
are still relevant. In fact, the performance hit on using getValue()
is much more severe here than for LazyConstraintCallback
, as we will pay the price at every node, not just at every integer solution.
Polynomial Time Separation for TSP over Cutset Constraints
Given a fraction solution , we need a polynomial time algorithm that will find the most violated cutset constraint (as previously defined here),
\[ \begin{aligned} && \sum_{e \in \delta(S)} x_e &\geq 2& S &\subset V&S&\neq V,\, \emptyset\\ \end{aligned} \]This is known as the separation problem. If we take each to be the weight of edge and form a weighted subgraph of the original graph where every node is present, every edge with is present, and the weights are given by the , then we can solve this problem by finding the global minimum cut in the graph. A wide range of algorithms have been devised to solve this problem, e.g.
- A single larger max flow min cut computation by Hao and Orlin,
- A randomized contraction algorithm by Karger and Stein
- A simple deterministic algorithm by Stoer and Wagner that has been implemented (although not optimally) in JGraphT
- A variety of more modern improvements by Karger (2000, 2009) and other authors.
- See also here for implementation details of some of these algorithms
Instead we will take a much simpler approach. Fix some node , and observe that in every cut must be on one side of the cut or the other. Thus it suffices to solve minimum cut problems, for each , . Then if is the minimum global cut, WLOG , then as , for some , when we compute the minimum cut, we will have , so the result will be the global minimum cut.
TODO add a graphic.
Implementing UserCutCallback for the Cutset Constraints
Much like with LazyConstraintCallback
, we will extend UserCutCallback
with an innner class inside TspIpSolver
. At the bottom of TspIpSolver.java (but before the final '}' ending the class), add the following code to extend UserCutCallback
:
private class MinCutCallback extends UserCutCallback{ private double minCutVal; public MinCutCallback(){ minCutVal = 1.99; } @Override protected void main() throws IloException { //fill this in } }
The value minCutVal is a numerical tolerance, we will only consider a constraint violated if the edges sum to less than 1.99 (the constraint says they should sum to at least 2.0). Next, we need to tell our solver to use the MinCutCallback
, but only the option Option.userCut
is selected. At the end of the constructor for TspIpSolver
, add
if(options.contains(Option.userCut)){ cplex.use(new MinCutCallback()); }
Recall previously that we defined a method getEdgesUsed()
that takes an array of doubles, one for each edge, where it is assumed that each edge value is either zero or one, and returns the subset of the edges taking the value one. We need an analagous method for one the edge weights are fractional. Add the following method to TspIpSolver
after getEdgesUsed()
private Map<E,Double> getNonZeroEdgeWeights(double[] edgeValues){ Map<E,Double> edgeWeights = new HashMap<E,Double>(); for(int i = 0; i < edgeValues.length; i++){ if(edgeValues[i] > Util.epsilon){ edgeWeights.put(edgeVariables.inverse().get(edgeVariablesAsArray[i]), edgeValues[i]); } } return edgeWeights; }
Given the edge weights, assumed to be in the order of the edge array edgeVariablesAsArray
, this method returns a Map taking edges to their weight, but edges with weight zero are excluded.
Now you need to fill in the method main()
from the callback, which should check to see if the current fractional solution violates any cutset constraints, and if so, add those constraints. Here is an outline of one possible solution:
- Exit (by returning) if we are not yet out of the "cut loop."
- Get a
double[]
of all the edge variable values - Convert this into a Map<E,Double> containing only those edges taking the value greater than zero.
- Form a subgraph of these edges.
- Fix a node , then for every other , compute the minimum cut.
- For every cut with weight less than
minCutVal
(1.99), find all edges across the cut (including edges with weight zero that were not in the subgraph) - For each of these edge sets, add a new cutset constraint to the model.
Code has been provided to perform step 3 with getNonZeroEdgeWeights()
and to aid in steps 4-6. In particular, we provide the class MinCutSolver
which has the constructor
Arguments |
Description |
---|---|
UndirectedGraph<V,E> graph, Map<E,Double> edgeWeights |
Takes in the entire graph, forms a subgraph containing only the edges where edgeWeights.get(edge).doubleValue()>0, calculates arbitrary minimum cuts |
and the method
Method Name |
Return Type |
Arguments |
Description |
---|---|---|---|
computeMinCut |
Set<V> |
V source, V sink |
Computes the minimum source-sink cut, and returns all nodes on the source side. |
The following methods and fields should all be useful
- edgeVariablesAsArray, from
TspIpSolver
which you can apply getValues() directly to. - cutEdges() from
TspInstance
- integerSum() from
Util
- calcSum() from
Util
Solution
Complete Source
Just in case, right now, TspIpSolver
should look something like this:
Show TspIpSolver